MonkeDonkeDev: C++ and Unreal Engine
Engine Version: 4.22.3
Introduction
In this tutorials I will show you:
-
How to create a dynamic multicast delegate in C++
-
How to execute it in C++, by using .Broadcast()
-
How to bind an event to it in Blueprint
Tutorial
In this example, I will create a C++ class EventCreator. Open your EventCreator.h file and create a dynamic multicast delegate on top of your header file, under the include statements and above UCLASS(). The first character of the name of the the delegate needs to be 'F'. Lets call it FTestEvent:
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FTestEvent);
After we have created the delegate, we declare it as a property in our EventCreator class. In the property specifiers list, we need to add BlueprintAssignable:
UPROPERTY(BlueprintAssignable);
FTestEvent OnTestEvent;
This makes sure that we can bind an event to the delegate in the blueprint editor. The code is shown below. (Ignore the STRINGTUTORIAL_API, it implies that the name of my project is stringtutorial, which I used in a previous tutorial)
After creating the declaration, we open our EvenCreator.cpp file, and execute it in the Beginplay function. To execute a delegate, we use Broadcast(). The code is shown below.
Now that we have set up our C++ code, we create a blueprint class that is based on this class. Lets call it BP_EventCreator. A blueprint class based on our C++ class can be created by right clicking on our C++ class in the content window in the editor and choose Create Blueprint class based on EventCreator.
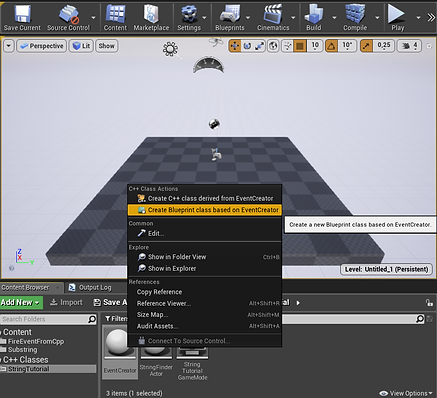
In our Event Graph, right-click on an empty space in the event graph and type the name of the delegate we created in our C++ code: OnTestEvent. We can see it appearing in the list.
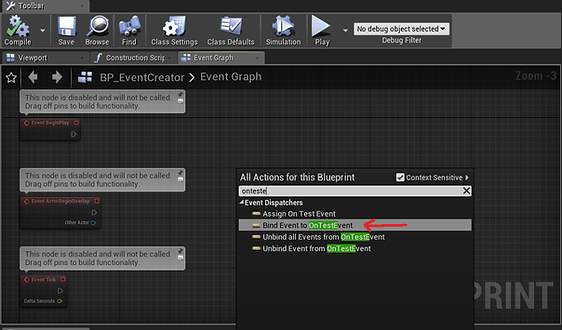
When we click on it, a Bind Event to node appears. We can bind any custom event to this node. Lets create a custom event named OnTestEvent and plug a Print String node to see if it executes. A custom event can be created by right-clicking on an empty space again and typing Custom Event. Finally, we bind this event to the Bind Event to node in our Event BeginPlay.
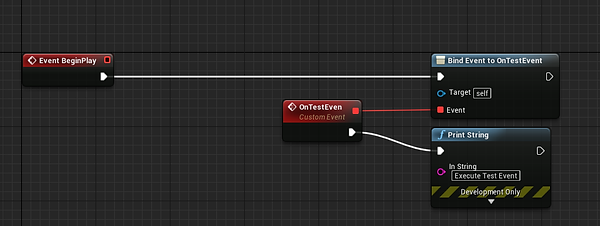
As we can see, the event executes and prints out Execute Teset Event.
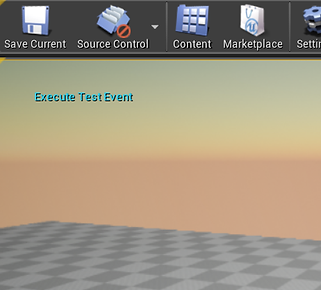
Voilà! I hope you enjoyed the tutorials and stay tuned for more!