MonkeDonkeDev: C++ and Unreal Engine
Engine Version: 4.22.3
Introduction
In this tutorial, I will show you how to find and extract text from a string by using FString::Find and FString::Mid.
FString is the way to go when string manipulation is desired, because FString is mutable as opposed to FName.
Tutorial
To show this example, I created an actor AStringFinderActor and put all the string operation in the Beginplay. This is only to easily print out and check the results.
Let's build up an example: we have cards, each one having a unique color and number. Every card has a format: Card_Color_Number, e.g. "Card_Red_56". Because we know the format of the card string, we can use it to our advantage.
Lets extract the color of the card, in this example it's "Red".
The first step is finding the index of the first end second '_' character. We will use FString::Find to find the index. The complete declaration of the function is shown below [1].
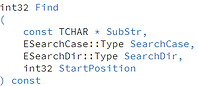
SubStr: the character you want to search in the string
Searchcase: if the search needs to be case sensitive or not. Choose CaseInsensitive when possible, because it is better performing.
SearchDir: indicates if the search starts from the beginning or the end of the string
StartPosition: the index from where it start to search the character
FString::Find and FString::Mid
A string is an array of characters, this means that each character has an index. In our example, it looks like this:

To find the index of the first '_', which is 4 in this case, we will use FString::Find. When the index is printed out to the output log, it shows indeed 4 as expected.
After we have found the first '_', we will find the index of the second '_' character, by passing the argument (index of first '_' + 1) to the StartPosition parameter in the parameter list of FString::Find. When the index of the second '_' character is printed out to the output log, it shows 8 as expected. The code is shown below.
Because we know the first and second index, we can determine the length of the color string inside our card string. We can determine the length by (indexOfSecondChar - indexOfFirstChar) - 1.
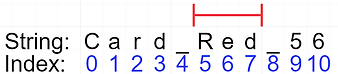
After we have the length, we can extract the color string by using the FString::Mid function. This function will extract a string inside a string, starting from a start index and counting from left to right. Complete declaration is shown below [2].
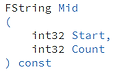
Start: start index where the extraction begins
Count: the number of character it will extract starting from Start
So we use FString::Mid by passing the argument (indexOfFirstCharacter + 1) to Start and LengthOfColor to Count. The code is shown below.
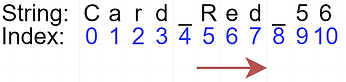
When we print all these values out, we can see that the color "Red" is extracted successfully.
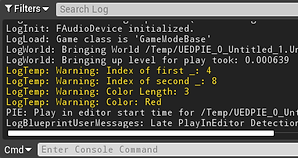
I hope you enjoyed this tutorial and stay tuned for more!
References
[1] https://docs.unrealengine.com/en-US/API/Runtime/Core/Containers/FString/Find/1/index.html
[2] https://docs.unrealengine.com/en-US/API/Runtime/Core/Containers/FString/Mid/index.html